「ChatGPTのCode Interpreterの活用方法がわからない…」「ChatGPTのCode Interpreterを使って画像の処理ができるの?」そう思う方も多いのではないでしょうか。
実は、ChatGPT Code Interpreterを活用することで、簡単に画像ファイルの処理を行うことができます。
今回PROMPTYでは、ChatGPT Code Interpreterを使った画像ファイルの処理方法を例を使ってご紹介します。
Code Interpreterの概要について知りたい方は、こちらの記事をご覧ください。
ChatGPTのCode Interpreterというコード実行プラグインがChatGPT Plus会員(有料会員)にリリースされたことが分かりました。 これにより、ChatGPTを使用している間、データ解析やグラフ生成といった機能[…]
① 画像のフォーマットを変更する
今回はこちらの画像の拡張子を変更して保存します。
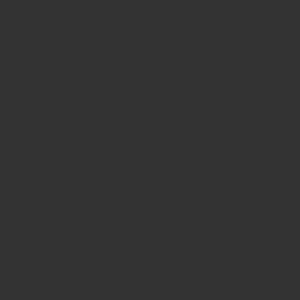
プロンプト:
この画像をpngファイルに変換して出力してください。
GPT-4の出力(Code Interpreter):
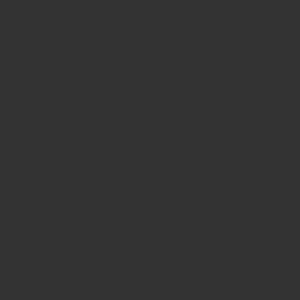
プロンプトの指示通り、jpegの画像をpngに変換してくれました。このように画像をアップロードして指示を与えるだけで、画像ファイルの処理を行うことができます。
Code Interpreterで実行したPythonコードはこちらです。
# Load the new image
img_new_3 = Image.open("/mnt/data/sample.jpeg")
# Save the image in PNG format
img_new_3.save("/mnt/data/sample_new_3.png")
"/mnt/data/sample_new_3.png"
② 画像をグレースケール(白黒)画像に変換する
プロンプト:
この画像をグレースケール画像に変換してください。変換後の画像を適切な名前で出力してください。
変換前の画像と並べて表示してください。
GPT-4の出力(Code Interpreter):
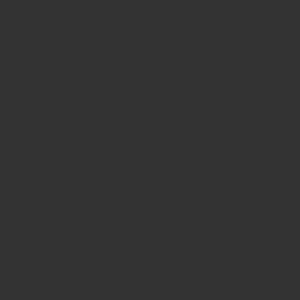
プロンプトの指示通りにRGB画像をグレースケール画像に変換してくれました。
変換前の画像並べて比較することもできています。
Code Interpreterで実行したPythonコードはこちらです。
# Load the new image
img_new_2 = Image.open("/mnt/data/sample.jpeg")
# Convert to grayscale
img_new_2_gray = img_new_2.convert("L")
# Save the grayscale image
img_new_2_gray.save("/mnt/data/sample_new_2_gray.jpeg")
# Display the original and grayscale images side by side
fig, ax = plt.subplots(1, 2, figsize=(12, 6))
# Original image
ax[0].imshow(img_new_2)
ax[0].set_title("Original Image")
ax[0].axis("off")
# Grayscale image
ax[1].imshow(img_new_2_gray, cmap='gray')
ax[1].set_title("Grayscale Image")
ax[1].axis("off")
plt.show()
③ テーマを指定して画像を処理する
プロンプト:
この画像をレトロ風に変換してください。変換後の画像を適切な名前で保存してください。
変換前の画像と並べて表示してください。
GPT-4の出力(Code Interpreter):
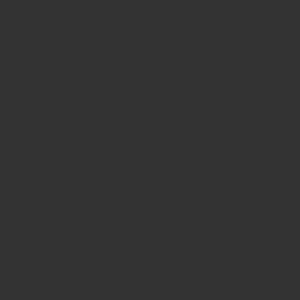
レトロ風という指示の意図を理解して、セピア色に変換しました。このように、テーマを与えるだけで、それに沿った処理を選んでくれることがChatGPT Code Interpreterの大きなメリットと考えられます。
Code Interpreterで実行したPythonコードはこちらです。
from PIL import ImageEnhance
# Load the new image
img_new_4 = Image.open("/mnt/data/sample.jpeg")
# Convert image to sepia
img_new_4 = img_new_4.convert("RGB")
sepia_image = Image.new("RGB", img_new_4.size)
sepia_list = []
for pixel in img_new_4.getdata():
r, g, b = pixel
# Sepia transformation
tr = int(0.393 * r + 0.769 * g + 0.189 * b)
tg = int(0.349 * r + 0.686 * g + 0.168 * b)
tb = int(0.272 * r + 0.534 * g + 0.131 * b)
if tr > 255: tr = 255
if tg > 255: tg = 255
if tb > 255: tb = 255
sepia_list.append((tr,tg,tb))
sepia_image.putdata(sepia_list)
# Save the retro-style image
sepia_image.save("/mnt/data/sample_new_4_retro.jpeg")
# Display the original and retro-style images side by side
fig, ax = plt.subplots(1, 2, figsize=(12, 6))
# Original image
ax[0].imshow(img_new_4)
ax[0].set_title("Original Image")
ax[0].axis("off")
# Retro-style image
ax[1].imshow(sepia_image)
ax[1].set_title("Retro-style Image")
ax[1].axis("off")
plt.show()
④ 物体の輪郭を検出する
今回はこちらの画像の輪郭を検出してもらいます。
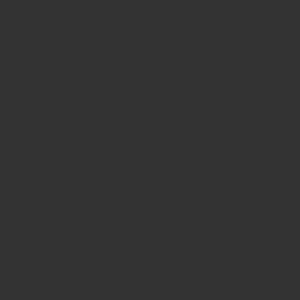
プロンプト:
この画像の輪郭を検出して、緑色で囲んでください。
GPT-4の出力(Code Interpreter):
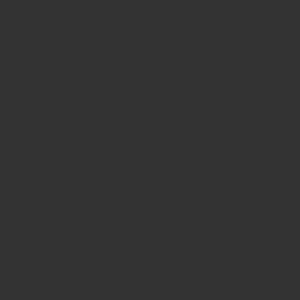
プロンプトの指示通り、食パンの輪郭を検出して、緑色で囲んだ画像が出力されました。
今回はCanny法をいう手法で輪郭検出を行っています。特に詳細な指示を出すことなく、最適な方法を選択して、輪郭の検出をしてくれました。
Code Interpreterで実行したPythonコードはこちらです。
import cv2
import numpy as np
# Load the image using OpenCV
img_new_6 = cv2.imread("/mnt/data/sample2.jpg")
# Convert to grayscale
gray = cv2.cvtColor(img_new_6, cv2.COLOR_BGR2GRAY)
# Perform edge detection
edges = cv2.Canny(gray, threshold1=30, threshold2=100)
# Perform a dilation and erosion to close gaps in between object edges
dilated_edges = cv2.dilate(edges, None, iterations=2)
edges = cv2.erode(dilated_edges, None, iterations=1)
# Find contours in the edge map
contours, _ = cv2.findContours(edges.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Draw contours
cv2.drawContours(img_new_6, contours, -1, (0,255,0), 2)
# Save the image with contours
cv2.imwrite("/mnt/data/sample_new_6_contours.jpg", img_new_6)
# Convert the color from BGR to RGB (for correct display in matplotlib)
img_new_6 = cv2.cvtColor(img_new_6, cv2.COLOR_BGR2RGB)
# Display the original image and the image with contours
fig, ax = plt.subplots(1, 2, figsize=(12, 6))
# Original image
ax[0].imshow(Image.open("/mnt/data/sample2.jpg"))
ax[0].set_title("Original Image")
ax[0].axis("off")
# Image with contours
ax[1].imshow(img_new_6)
ax[1].set_title("Image with Contours")
ax[1].axis("off")
plt.show()
まとめ
今回PROMPTYでは、ChatGPT Code Interpreterを活用して、次のような画像ファイルの処理方法を紹介しました。
- 画像のフォーマットを変更する
- RGB画像をグレースケールに変換する
- テーマを指定して、それに沿った画像の処理を行う
- 物体の輪郭を検出する
画像をアップロードして、日本語で指示を出すだけで、所望の画像ファイル処理ができることが、ChatGPT Code Interpreterの大きな強みと言えるでしょう。
今回の例を参考に、皆さまもChatGPT Code Interpreterを使った画像ファイルの処理を試してみてはいかがでしょうか。